Risk engine
Introduction
The risk engine is a low-code interface that helps you define and apply your risk assessment rules to your cases.
The key concept to understand:
-
Deployment status and versioning : when building on a risk engine, it will be auto-saved as Draft until you publish it and turn it online. Once it’s published, the versioning will start, and all updates that you save and publish afterwards will be stored as previous versions.
-
Multiple risk engine : You can have multiple risk engines online at the same time. This is for example when you have very different risk assessment rules for different types of cases (KYB and KYC cases for instance). In this case, it’s possible to build a routing logic on each risk engine to make sure the relevant online one is applied.
Access the inputs
The inputs for your risk engines must be data centralized inside Dotfile. These inputs can be retrieved using predefined JavaScript methods.
$.input.case
: Access the Case object$.input.companies
: Access an array of the companies of the Case$.input.individuals
: Access an array of the individuals of the Case$.input.companies.checks
or$.input.individuals.checks
: Access an array of checks of a company or an individual
Examples :
// Isolate the main company
const mainCompany = $.input.companies.find((x) => x.type === "main");
// Isolate the beneficial owners
const UBOs = $.input.individuals.filter((x) => x.is_beneficial_owner);
Use Lookup Tables
Lookup tables enable you to map inputs for your Risk Engine, which is particularly useful for defining mappings such as country or industry risk levels. For instance, you can assign different risk levels or scores to properties with multiple option values based on your specific criteria.
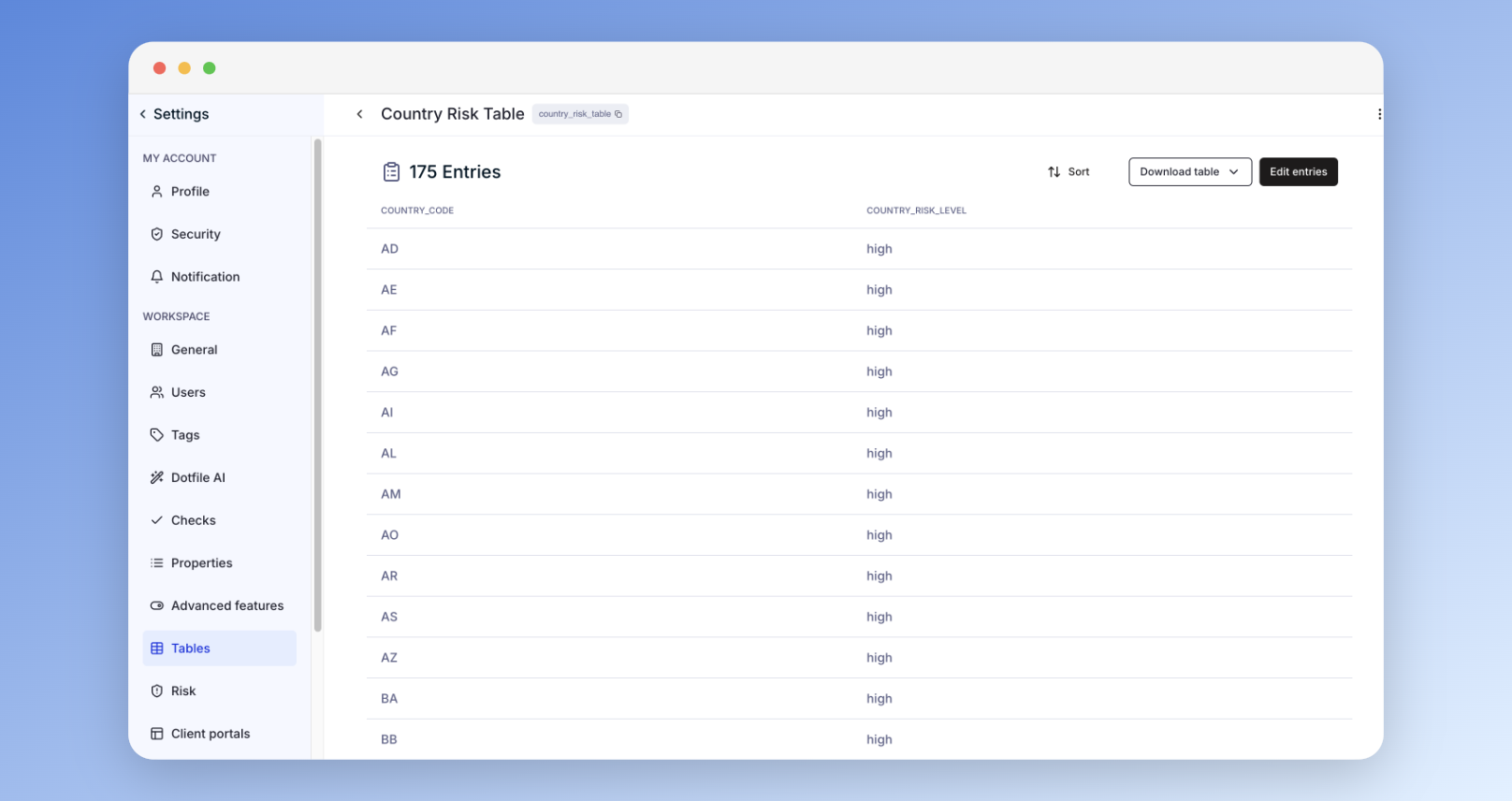
How to Create a Lookup Table :
- Navigate to Settings and open the Tables tab.
- Upload your table file in one of the supported formats:
.csv
,.xls
, or.xlsx
Once uploaded, these tables can be referenced in your risk assessment rules using the predefined method $.lookup()
Example :
const mainCompany = $.input.companies.find((x) => x.type === "main");
const main_company_country = mainCompany?.country;
const country_of_registration_risk = main_company_country
? $.lookup({
table_key: "country_risk_table",
lookup_column: "country_code",
lookup_value: main_company_country,
output_column: "country_risk_level",
}) ?? "not_defined"
: "not_defined";
Use the predefined methods
Method | Description |
---|---|
$.lookup() | Lookup a value of a Table in the risk engine :$.lookup(lookup_object_input) Lookup Object Parameters: - table_key : string — The key of the table to look up.- lookup_column : string — The key of the header column to match with the lookup_value.- lookup_value : string — The value to match within the lookup_column.- output_column : string — The key of the header column to return the value from, if a match is found.- output_type : optional — Specifies the type of the output. Can be one of the following: string (default), Boolean , Number Return Type: null | T — Where he type T is inferred based on the output_type .- Returns null if no matching row is found for the given lookup_value in the lookup_column .- Returns the matched value, optionally cast to boolean or number based on the specified output_type . |
$.set_risk() | Set the risk on the case and stop the execution of the code :$.set_risk(risk_object) Parameters for risk_object : - level : The risk level to be assigned to the case.- score : optional — A number or null representing the risk score.- components : optional — An object with string keys and number values, representing the individual components of the risk. Default is null.- flags : optional — An object with string keys and boolean values, used to indicate flags related to the risk. Default is null.- comment : optional — A string or null, allowing you to add an optional comment on the risk. |
$.exit() | Stops the execution of the code |
Risk Engine Example
// Inputs
const beneficial_owners = $.input.individuals.filter(
(x) => x.is_beneficial_owner,
);
const main_company = $.input.companies.find((x) => x.type === "main");
// Helpers
function ageInYears(date) {
date = new Date(date);
const today = new Date();
const difference = today.getTime() - date.getTime();
return difference / (1000 * 3600 * 24 * 365);
}
// Score components
const prohibited_risk_flags = {};
const high_risk_flags = {};
if (!main_company) $.exit();
let maturity_score = 100;
if (main_company.registration_date) {
const age = ageInYears(main_company.registration_date);
if (age > 2) maturity_score = 50;
}
let registration_country_score = 100;
if (main_company.country) {
const found_score = $.lookup({
table_key: "countries",
lookup_column: "country",
lookup_value: main_company.country,
output_column: "score",
output_type: "number",
});
const found_risk = $.lookup({
table_key: "countries",
lookup_column: "country",
lookup_value: main_company.country,
output_column: "risk",
});
if (typeof found_score === "number") registration_country_score = found_score;
if (found_risk === "High") high_risk_flags["High risk country"] = true;
}
const components = {
"Maturity score": maturity_score,
"Registration country score": registration_country_score,
};
// Final Score
const score = Math.ceil(
maturity_score * 0.2 + registration_country_score * 0.5,
);
const level =
Object.keys(prohibited_risk_flags).length > 0
? "prohibited"
: score >= 2200 || Object.keys(high_risk_flags).length > 0
? "high"
: score < 1800
? "low"
: "medium";
$.set_risk({
level,
score,
components,
flags: { ...high_risk_flags, ...prohibited_risk_flags },
});
Automated risk via API
If you wish to manage the risk assessment on your side, you can use our dedicated API endpoint to set the risk score and its different components.
Using this endpoint in combination with our webhooks will allow you to recompute the risk whenever changes on the case and its entities occur. More information can be found here.
Support and Services for Risk Engine Deployment
The Dotfile team is here to support you in deploying your risk engine. We’ll provide technical guidance and run workshops to help you implement your custom risk model based on your input, such as an Excel or Google Sheet.
Your risk engine should leverage centralized data in Dotfile, including data collected through the Client Portal, manual inputs, or external sources integrated via API. If you need to adjust the risk assessment rules, our team is available to guide you and support updates effectively.
Updated 5 months ago