Workflows
Introduction
The Workflow feature is an alternative to Templates when it comes to determining which checks will be created for which entity. It allows you to implement check creation rules with JavaScript code.
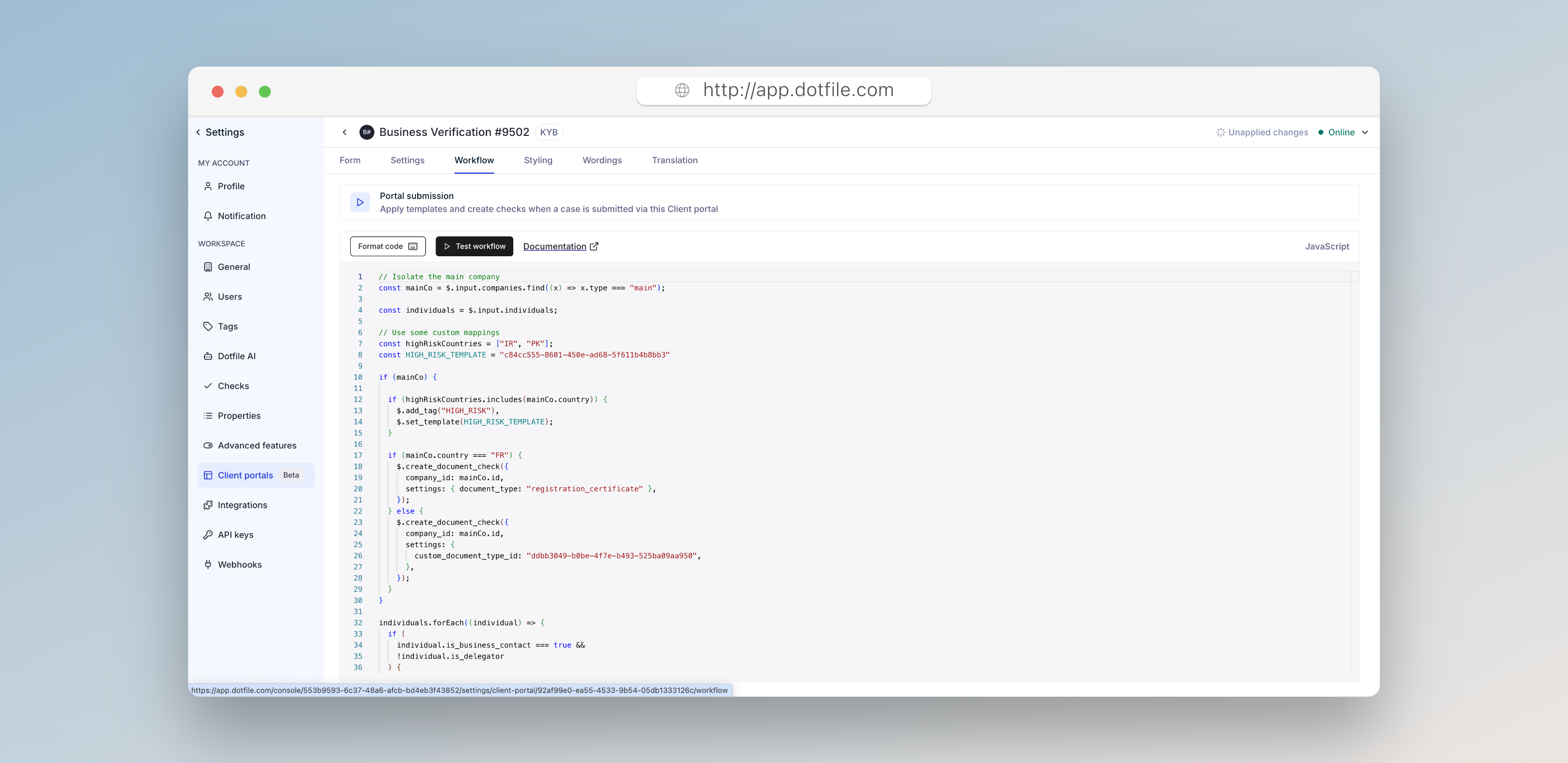
Use Case Examples
- Dynamically set the template or create checks based on advanced conditions:
- Apply the template named “Non-European Countries” to any company not based in the EU.
- Request a “Power of Attorney” document check from a business contact if they are not a legal representative of the company, and also request an ID document check from the person who granted power to the business contact.
- Add tags to the case under specific conditions:
- Add a tag to the KYC case if the individual is above a certain age.
Access the objects
$.input.case
Access the Case object$.input.companies
Access an array of the companies of the Case$.input.individuals
Access an array of the individuals of the Case$.input.companies.checks
or$.input.individuals.checks
: Access an array of checks of a company or an individual$.input.case.risk
: Access the current case object of the case
It's easy to filter those objects based on your own business rules
// Isolate the main company
const mainCo = $.input.companies.find((x) => x.type === "main");
// Isolate the beneficial owners
const UBOs = $.input.individuals.filter((x) => x.is_beneficial_owner);
Use the predefined methods
Method | Description |
---|---|
$.add_tag(tagName|tagUUID) | Add a tag to the Case$.add_tag("HIGH_RISK") using existing tag name,$.add_tag("5e2750e5-7376-443e-9fe3-ab9e5aa4ff80") using existing tag UUID |
$.set_template(templateUUID) | Set template in a Case. This will override any template that would have been already set via the Client Portal settings.$.set_template("2cf878de-3b7c-414a-ae0d-61328ff2740b") |
$.create_aml_check() | $.create_document_check({ company_id: "XX", settings }) $.create_aml_check({ individual_id: "XX", settings }) Available settings https://docs.dotfile.com/reference/aml-create-one |
$.create_document_check() | $.create_document_check({ company_id: "XX", settings }) $.create_document_check({ individual_id: "XX", settings }) Available settings https://docs.dotfile.com/reference/document-create-one |
$.create_id_document_check() | $.create_document_check({ company_id: "XX", settings }) Available settings https://docs.dotfile.com/reference/id-document-create-one |
$.create_id_verification_check() | Available settings https://docs.dotfile.com/reference/id-verification-create-one |
Code Examples
Add a custom document check for all individuals that are beneficial owners
const UBOs = $.input.individuals.filter((x) => x.is_beneficial_owner);
UBOs.forEach((ubo) => {
$.create_document_check({
individual_id: UBOs.id,
settings: {
document_type_key: "9ff5c066-7446-4045-a453-6d4832bea585",
},
});
}
});
Add a native document check registration_certificate
for the main company and enable some automatic document analysis
const mainCo = $.input.companies.find((x) => x.type === "main");
$.create_document_check({
company_id: mainCo.id,
settings: {
document_analysis: {
automatic_rejection: true,
automatic_approval: true,
parameters: {
model: "registration_certificate",
authorized_documents: ["kbis"],
max_age_in_days: 30,
},
},
document_type: "registration_certificate",
},
});
Real life example
// Isolate the main company
const mainCo = $.input.companies.find((x) => x.type === "main");
const individuals = $.input.individuals;
// Use some custom mappings
const highRiskCountries = ["IR", "PK"];
const HIGH_RISK_TEMPLATE = "c84cc555-8601-450e-ad68-5f611b4b8bb3"
if (mainCo) {
if (highRiskCountries.includes(mainCo.country)) {
$.add_tag("HIGH_RISK"),
$.set_template(HIGH_RISK_TEMPLATE);
}
if (mainCo.country === "FR") {
$.create_document_check({
company_id: mainCo.id,
settings: { document_type: "registration_certificate" },
});
} else {
$.create_document_check({
company_id: mainCo.id,
settings: {
document_type_key: "ddbb3049-b0be-4f7e-b493-525ba09aa950",
},
});
}
}
individuals.forEach((individual) => {
if (
individual.is_business_contact === true &&
!individual.is_delegator
) {
$.create_document_check({
individual_id: individual.id,
settings: {
document_type_key: "9ff5c066-7446-4045-a453-6d4832bea585",
},
});
}
if (individual.is_business_contact === false && individual.is_delegator) {
$.create_id_document_check({
individual_id: individual.id,
});
}
});
Test your workflows
You can test that everything works as planned with your workflow by using existing cases. No actions will actually be performed, but you will be able to see what would have been executed based on the rules you defined.
Updated about 2 months ago